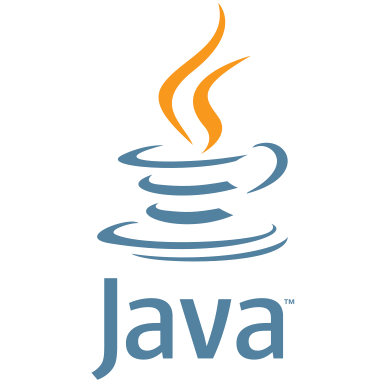
Java Tutorial for Beginners
Java is one of the top programming languages due to its simplicity, platform independence, robustness, and versatility. It is used for developing a wide range of applications, from desktop software to mobile apps and enterprise systems. If you are keen to learn this language, then our Java Tutorial is the perfect way to get started!
This tutorial on Java covers all the core topics and is perfect for anyone who is just starting out with programming.
Throughout the Java tutorial for beginners, we will provide clear explanations, code examples, and exercises to help you build your skills and knowledge in Java. By the end of this tutorial, you will have a solid understanding of Java programming and be able to create your own Java programs. So, let's get started and begin your journey into Java programming!
Introduction to Java
- What is Java Language? Definition, Uses, Features, Introduction
- Top 14 Features of Java Programming Language (Full List)
- History of Java (Evolution, Founder, All Versions of Java Language)
- Java Uses (Top 10 Uses & Applications of Java Programming)
- Basics of Java Programming (Fundamental Concepts of Java Language)
- All Editions of Java and Types (Standard, Enterprise, Micro)
- What is JVM (Java Virtual Machine)? Architecture, Components, Working
- Java Development Kit (JDK): Architecture, Components, Download
- Java Runtime Environment (JRE): Uses, Components, Download
- How & Why is Java Platform Independent? 7 Reasons
- Program Compilation in Java (How Java Programs Run and Execute?)
- Java Keywords List (With Use and Examples)
- What Are Java Comments? Types, Examples, Best Practices
- What Are Tokens in Java? All Types of Java Tokens
Control Flow and Decision Making
- Java Identifiers: Use, Examples, Rules, Valid/Invalid Identifiers
- What Are Variables in Java? Types, Examples, Declare, Initialize
- Java Constants: Example, Use, How to Declare Constant in Java?
- What Are Operators in Java? All Types of Java Operators
- Compound Assignment Operators in Java (With Examples)
- Java Arithmetic Operators (All Operators With Examples)
- Bitwise Operators in Java: AND, OR, XOR, Complement, Shift (With Examples)
- Java Logical Operators (AND, OR, NOT) With Examples
- All Java Relational Operators (Explained With Examples)
- What Are Loops in Java? For loop, while loop, do while loop (All Types)
- Java Conditional Statements: if, if-else, nested if, ladder, switch
- Nested Loop in Java (Nested for, while, do-while loops)
- for-each Loop in Java (Syntax, Examples, Flowchart)
- Java Continue Statement: Use, Examples, Jump Statements
- All Java Assignment Operators (Explained With Examples)
- Java Break Statement: Uses, Syntax, Examples, Label Break
Arrays and Collections
- What are Arrays in Java? Create, Types, Examples, Full Guide
- String Array in Java: Create, Declare, Initialize, Sort, Iterate, Examples
- Java Multidimensional Array (2D & 3D Arrays With Examples)
- What Are Java Collections? Java Framework Collection, Examples, Hierarchy
- What is Java Vector? Example, Use, Features, Vector Methods
Strings and Methods
- What is String in Java? How to Create Java String?
- How to Compare Strings in Java? String Comparison in Java (With Examples)
- Methods in Java: Definition, Types, Examples, Declaration
- Static Method in Java: Use, Examples, How to Declare and Call
- What is Method Overloading in Java? Explained With Example
- What is Method Overriding in Java? Examples, Uses, Rules
Java Exception Handling
- Exceptions in Java: Types, Hierarchy, Examples, Keywords
- Java Exception Handling: Mechanism, Keywords, Examples
- Java Null Pointer Exception: How to Avoid and Handle nullpointerexception?
- Java Custom Exception (User-Defined): Use, Examples, Create, Program
- Difference Between Throw and Throws in Java (With Examples)
- What is try catch in Java? Explained With Examples
- Try With Resources in Java (Syntax, Example, Benefits)
Threads and Multithreading in Java
Object-Oriented Programming in Java
- OOPs Concepts in Java (Explained With Examples)
- Classes and Objects in Java
- Java Instance Variable: Use, Features, Instance vs Class Variable
- What is Inheritance in Java? Examples, Types, Benefits, Use
- Java Abstract Class and Abstract Methods
- Java Interface: Definition, Example, Syntax, Implementation
- What is Polymorphism in Java? Example, Types, Use, Program
- What is Java Encapsulation? Example, Use, Benefits, Types, How to Achieve
Important Concepts
- ‘this’ Keyword in Java: Explained With Uses & Examples
- Java Logging & Loggers (Examples, Components, Levels, Info)
- Java Annotations: Use, Example, Types, Custom Annotation
- Enumeration in Java (Examples, Uses, Enum Class, Enumerators)
- Java Type Casting and Type Conversion (All Types With Examples)
- Java Expressions: Meaning, Types, Evaluation of Expression, Examples
- Java Data Types: Primitive & Non-Primitive With Examples
- Java Access Modifiers (Specifiers): Types, Examples, Uses
- Java Static Keyword: Use, Examples, Meaning
- What Are Constructors in Java? Types, Examples, Uses, Syntax
- Java Inner Class (Examples, Types, Benefits)
- Java Buzzwords (With Meaning and Examples)
- Java Anonymous (Inner) Class: Explained With Types, Examples
- Java Static Class (Explained With Examples)
- Java Super Keyword: Use, Examples, Benefits
- Java Final Keyword With Example (Variable, Method, Class)
- Java Singleton Class: Use, Example, How to Create (Full Guide)
- Java Assert Keyword & Assertion (Explained With Examples)
- Java Reflection: Meaning, Uses, Examples, Reflection API
- Java Serialization and Deserialization: Differences & Examples
- Java File Handling: Operations, Examples, Errors, Best Practices
- Streams in Java: Stream Operations & Functional Interfaces With Examples
- Java Recursion: Meaning, Types, Examples, Programs
- What Are Design Patterns in Java? Types, Benefits, Structure, Examples
- JavaBeans Class in Java: Properties, Examples, Benefits, Life Cycle
- What is Java Framework? Top Frameworks in Java
Learning Resources
- Top 44 Java String Interview Questions for Freshers to Experienced (2025)
- Top 30 Java Serialization Interview Questions & Answers
- Top 50 Java Exception Handling Interview Questions and Answers (2024)
- Top 45 Java Array Interview Questions and Answers(2025)
- Top 50 Java Multithreading Interview Questions and Answers